设计模式之组合模式
意图
组合模式 (Component) 是一种结构型设计模式,将对象组合成树形结构以表示“部分-整体”的层次结构。
组合模式使得用户对单个对象和组合对象的使用具有唯一性。
适用场景
组合模式的适用场景:
- 想要表示对象的部分-整体层次结构。
- 想要客户端忽略组合对象与单个对象的差异,客户端将统一地使用组合结构中的所有对象。
关于分级数据结构的一个普遍性的例子是你每次使用电脑时所遇到的 文件系统。
文件系统由目录和文件组成。每个目录都可以装内容。目录的内容可以是文件,也 可以是目录。
按照这种方式,计算机的文件系统就是以递归结构来组织的。如果你想要描述这样的数据结构,那么你可以使用组合模式。
结构
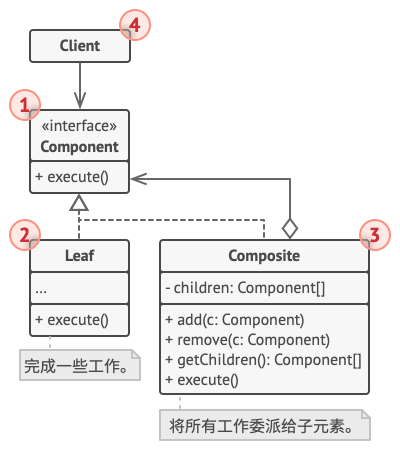
结构说明
- 组件 (Component) 接口描述了树中简单项目和复杂项目所共有的操作。
- 叶节点 (Leaf) 是树的基本结构, 它不包含子项目。一般情况下, 叶节点最终会完成大部分的实际工作, 因为它们无法将工作指派给其他部分。
- 容器 (Container)——又名 “组合 (Composite)”——是包含叶节点或其他容器等子项目的单位。 容器不知道其子项目所属的具体类, 它只通过通用的组件接口与其子项目交互。容器接收到请求后会将工作分配给自己的子项目, 处理中间结果, 然后将最终结果返回给客户端。
- 客户端 (Client) 通过组件接口与所有项目交互。 因此, 客户端能以相同方式与树状结构中的简单或复杂项目交互。
结构代码范式
Component : 组合中的对象声明接口,在适当的情况下,实现所有类共有接口的默认行为。声明一个接口用于访问和管理 Component 的子部件。
1 2 3 4 5 6 7 8 9 10 11
| abstract class Component { protected String name;
public Component(String name) { this.name = name; }
public abstract void Add(Component c); public abstract void Remove(Component c); public abstract void Display(int depth); }
|
Leaf : 表示叶节点对象。叶子节点没有子节点。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Leaf extends Component {
public Leaf(String name) { super(name); }
@Override public void Add(Component c) { System.out.println("Can not add to a leaf"); }
@Override public void Remove(Component c) { System.out.println("Can not remove from a leaf"); }
@Override public void Display(int depth) { String temp = ""; for (int i = 0; i < depth; i++) temp += '-'; System.out.println(temp + name); }
}
|
Composite : 定义枝节点行为,用来存储子部件,在 Component 接口中实现与子部件相关的操作。例如 Add 和 Remove。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| class Composite extends Component {
private List<Component> children = new ArrayList<Component>();
public Composite(String name) { super(name); }
@Override public void Add(Component c) { children.add(c); }
@Override public void Remove(Component c) { children.remove(c); }
@Override public void Display(int depth) { String temp = ""; for (int i = 0; i < depth; i++) temp += '-'; System.out.println(temp + name);
for (Component c : children) { c.Display(depth + 2); } }
}
|
Client : 通过 Component 接口操作结构中的对象。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public class CompositePattern {
public static void main(String[] args) { Composite root = new Composite("root"); root.Add(new Leaf("Leaf A")); root.Add(new Leaf("Leaf B"));
Composite compX = new Composite("Composite X"); compX.Add(new Leaf("Leaf XA")); compX.Add(new Leaf("Leaf XB")); root.Add(compX);
Composite compXY = new Composite("Composite XY"); compXY.Add(new Leaf("Leaf XYA")); compXY.Add(new Leaf("Leaf XYB")); compX.Add(compXY);
root.Display(1); }
}
|
伪代码
在本例中, 我们将借助组合模式帮助你在图形编辑器中实现一系列的几何图形。
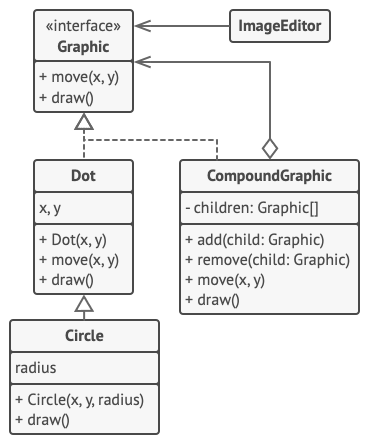
组合图形
CompoundGraphic 是一个容器, 它可以由多个包括容器在内的子图形构成。 组合图形与简单图形拥有相同的方法。 但是, 组合图形自身并不完成具体工作, 而是将请求递归地传递给自己的子项目, 然后 “汇总” 结果。
通过所有图形类所共有的接口, 客户端代码可以与所有图形互动。 因此, 客户端不知道与其交互的是简单图形还是组合图形。 客户端可以与非常复杂的对象结构进行交互, 而无需与组成该结构的实体类紧密耦合。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73
| interface Graphic is method move(x, y) method draw()
class Dot implements Graphic is field x, y
constructor Dot(x, y) { ... }
method move(x, y) is this.x += x, this.y += y
method draw() is
class Circle extends Dot is field radius
constructor Circle(x, y, radius) { ... }
method draw() is
class CompoundGraphic implements Graphic is field children: array of Graphic
method add(child: Graphic) is
method remove(child: Graphic) is
method move(x, y) is foreach (child in children) do child.move(x, y)
method draw() is
class ImageEditor is field all: CompoundGraphic
method load() is all = new CompoundGraphic() all.add(new Dot(1, 2)) all.add(new Circle(5, 3, 10))
method groupSelected(components: array of Graphic) is group = new CompoundGraphic() foreach (component in components) do group.add(component) all.remove(component) all.add(group) all.draw()
|
案例
使用实例: 组合模式在 Java 代码中很常见,常用于表示与图形打交道的用户界面组件或代码的层次结构。
下面是一些来自 Java 标准程序库中的组合示例:
识别方法: 组合可以通过将同一抽象或接口类型的实例放入树状结构的行为方法来轻松识别。
与其他模式的关系
- 桥接模式、 状态模式和策略模式 (在某种程度上包括适配器模式) 模式的接口非常相似。 实际上, 它们都基于组合模式——即将工作委派给其他对象, 不过也各自解决了不同的问题。 模式并不只是以特定方式组织代码的配方, 你还可以使用它们来和其他开发者讨论模式所解决的问题。
- 你可以在创建复杂组合树时使用生成器模式, 因为这可使其构造步骤以递归的方式运行。
- 责任链模式通常和组合模式结合使用。 在这种情况下, 叶组件接收到请求后, 可以将请求沿包含全体父组件的链一直传递至对象树的底部。
- 你可以使用迭代器模式来遍历组合树。
- 你可以使用访问者模式对整个组合树执行操作。
- 你可以使用享元模式实现组合树的共享叶节点以节省内存。
- 组合和装饰模式的结构图很相似, 因为两者都依赖递归组合来组织无限数量的对象。
- 装饰类似于组合, 但其只有一个子组件。 此外还有一个明显不同: 装饰为被封装对象添加了额外的职责, 组合仅对其子节点的结果进行了 “求和”。
- 但是, 模式也可以相互合作: 你可以使用装饰来扩展组合树中特定对象的行为。
- 大量使用组合和装饰的设计通常可从对于原型模式的使用中获益。 你可以通过该模式来复制复杂结构, 而非从零开始重新构造。
参考资料